Data Streams – A convenient way to read-write primitives in Java
|Similar to the Character Streams that makes it easy to read and write character files Data Streams are used to perform binary input output operations of all the primitive data types in Java including Strings i.e. if you want to perform i/o operations for boolean, char, byte, short, int, long, float, double or Strings then data streams provide a very convenient and an efficient way for that. This allows you to read-write Java primitives instead of raw bytes ( Isn’t that cool 🙂 ).
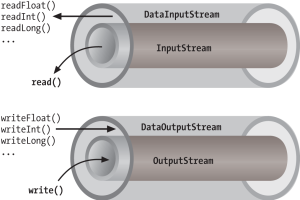
Interfaces
Every Data Stream class implements either of the two interfaces-
Important classes
A lot of classes implements these interfaces but here we are gonna talk about those 2 classes which are most widely used. These are-
DataInputStream
- It wraps an InputStream Object and let the application read the Java primitive data types from the wrapped input stream.
- This whole process of reading and converting into a primitive data is machine independent.
- User is responsible for making DataInputStream safe in multithreaded environment.
- DataOutputStream is used to write data which is used by DataInputStream to read later.
DataOutputStream
- It wraps an OutputStream Object and let the application write Java primitives to the wrapped output stream.
- DataInputStream is used to read back the data written by this data stream.
Note:- Each method of DataOutputStream is matched with corresponding method of DataInputStream. Be assure that you are using the corresponding method and in the correct order otherwise while reading data, output will be unexpected. Example – readDouble() for writeDouble( double ), readUTF() for writeUTF(String) etc.
Note:- Since we generally use Buffered Streams for improving performance either we should us try with resources or we should close the stream or at least flush it in case of Output Stream otherwise we could lose the buffered data.
Note:- Read comments on code carefully.
Example – Writing data to file using DataOutputStream
package codingeekExamples; import java.io.*; public class DataOutputStreamExample { static final double[] price = { 15.43, 10.12, 50.8, 249.56, 18.99 }; static final int[] qty = { 10, 23, 45, 14, 2 }; static final String[] items = { "keyboard", "mouse", "core java ebook", "ups", "pen drive", }; public static void main(String[] args) { /** * Use try with resources or don't forget to close the stream( because * we use BufferedStream here). */ try ( // Create File output Stream. data.txt should be in classpath or provide fully qualified path OutputStream os = new FileOutputStream("data.txt"); // Create Buffered stream. You can skip this step also BufferedOutputStream bos = new BufferedOutputStream(os); // create data output stream DataOutputStream out = new DataOutputStream(bos) ) { // stuffing item name, quantity, price one by one in output stream for (int i = 0; i < price.length; i++) { out.writeUTF(items[i]); out.writeInt(qty[i]); out.writeDouble(price[i]); } System.out.println("Data successfully written to a file"); } catch (IOException e) { e.printStackTrace(); } } }
Output:-
Data successfully written to a file
Example – Reading data from a file using DataInputStream
package codingeekExamples; import java.io.*; public class DataInputStreamExample { public static void main(String[] args) { double price; int quantity; String item; try ( // create input stream. Same rules applies to file name again InputStream is = new FileInputStream("data.txt"); // create buffered input stream. you can skip this. BufferedInputStream bis = new BufferedInputStream(is); // create data input stream to read data in form of primitives. DataInputStream in = new DataInputStream(bis);) { System.out.println("Selected items are"); /** * Reading whole data from the file. Remember to read it in the same * order in which it was stored i.e. if integer is stored first then * readInt() method should be invoked first otherwise unexpected * error or output will be there. */ while (in.available() > 0) { item = in.readUTF(); quantity = in.readInt(); price = in.readDouble(); System.out.format("%-20s %-10d $%.2f%n", item, quantity, price); } } catch (FileNotFoundException e) { e.printStackTrace(); } catch (IOException e) { e.printStackTrace(); } } }
Output:-
Selected items are
keyboard 10 $15.43
mouse 23 $10.12
core java ebook 45 $50.80
ups 14 $249.56
pen drive 2 $18.99
Source:- Oracle
Hi Hitesh,It is bit helpful, but actually what i have required that i need to read the stream from stream buffer and store it on hash table can u help me on.
Note: stream is being generated through stram generator an need to be read by parts of 75 records at a time.
Hi Sajid,
Could you please use http://www.codingeek.com/forum to ask this question and provide some details as it will help to understand your questions better.
Please elaborate and provide the code in which you are facing the issue.
I will definitely try to answer your issue.