Objects and Classes in Java
|In this I am gonna discuss how objects and classes are related, how to use them and what is their relation. But before starting make sure you know about the concepts of OOPS(atleast read how Steve Jobs describe OOPS).
So as we all know that oops revolve around the object.. So what the hell are these objects??
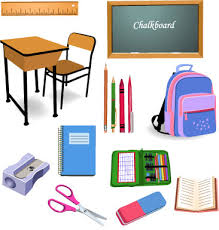
Objects are the instances of our classes. In any object oriented language classes acts as the blueprint of the object i.e. a Class is just a written piece of code while an object is the real implementation of it. All the variables that we declare inside a class became the attributes and all the methods becomes the behaviour of the created object.
Lets take a working example of it using Java..
A class in java can contain-
- data member
- method
- constructor
- block
- class and interface
class { data members; constructors; methods; Inner_classes or interfaces; }
A Simple Example of object and Class
class Employee{ //id and company data member(also instance variable) int id; String company; public static void main(String args[]){ Employee employee=new Employee();//creating an object of Employee System.out.println(employee.id+" "+employee.company); } }
0 null
In the above example we see that
- We create two instance variables( these are the variables that are accessible in the whole class) i.e id and company.
- Then we create a main (String[] arg) method which is static and public which ensures that this is the entry point of the program.
- Then we create an object of Employee class with reference “employee” which creates an object in the heap and its address is stored in the variable “employee” which is implicitly stored in stack.
- finally we print the value of emplpyee id and his company but we get get some unexpected output i.e. (id->0 and comapny -> null). this is because when we create an object in java it automatically allocates the defalut value to all of its variables and hence we got ‘0’ as integer default value and “null” and a string’s default value.
So let’s see an example how to fix this and how to set values in a java program and then access it using the same method.
class Employee{ int id; String company; public static void main(String args[]){ Employee employee=new Employee();//creating an object of Employee employee.id=100; // Setting value of employee id employee.company = "Oracle"; // Setting value of Employee company System.out.println(employee.id+" "+employee.company); } }
100 Oracle
So we can see that as soon as we set the properties of employee and when we access them we are getting the expected values.
Also we can make any number of objects or can use the same reference variable again and again to set the different values and use them at different places.
Checkout this post to see what are the different ways of creating an object and how to use methods and parameterised constructors.